How to use React Context effectively

- Published on
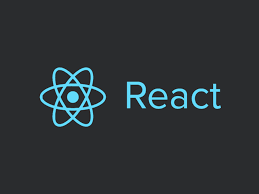
Effectively Utilizing React Context
React Context is a powerful tool for managing state and sharing data across a React application. It provides a way to pass data without explicitly passing props down through every level of the component tree. This can simplify code and make it easier to manage complex state.
When to Use React Context
React Context is particularly useful in situations where:
State needs to be shared across multiple components: If you have a piece of state that needs to be accessed by multiple components that are not directly related to each other, React Context can provide a more elegant solution than prop drilling.
State needs to be global: If you have a truly global state that needs to be accessible throughout the application, React Context can be a good choice. However, it's important to use global state sparingly and thoughtfully, as it can make the application more difficult to reason about and maintain.
Creating a Context
To create a context, you'll need to use the React.createContext()
function. This function takes a default value as an argument, which will be used if no provider is found.
import React from 'react';
const MyContext = React.createContext(defaultValue);
Providing Context
Once you've created a context, you need to provide it to your components using a Context.Provider
component. The Context.Provider
component takes a value prop that will be made available to any child components that are descendants of the Context.Provider
.
import React from 'react';
import MyContext from './MyContext';
const MyComponent = () => {
const value = useContext(MyContext);
return <div>MyContext value: {value}</div>;
};
const App = () => {
return (
<MyContext.Provider value="Hello from Context">
<MyComponent />
</MyContext.Provider>
);
};
Consuming Context
To consume a context, you can use the useContext()
hook. The useContext()
hook takes a context as an argument and returns the value provided by the nearest Context.Provider
ancestor of the current component.
import React from 'react';
import MyContext from './MyContext';
const MyComponent = () => {
const value = useContext(MyContext);
return <div>MyContext value: {value}</div>;
};
Best Practices for Using React Context
Here are some best practices for using React Context effectively:
Use Context sparingly: Only use context when it's truly needed. Overusing context can make your application more difficult to understand and maintain.
Name your contexts thoughtfully: Use descriptive names for your contexts so that it's easy to understand what they represent.
Avoid prop drilling: Use context to avoid passing props down through multiple levels of the component tree.
Memoize components: Memoize components that consume context to prevent unnecessary re-renders when the context value doesn't change.
Use context for shared state, not business logic: Context is for sharing state, not for implementing business logic. Keep your business logic in separate components.
Consider using alternative state management solutions: If your application has complex state management needs, consider using a more robust state management library, such as Redux or MobX.
By following these best practices, you can effectively utilize React Context to manage state and share data across your React applications.